
Originally Posted by
Megahertz
1) Can you please explain how is "Start reading average pressure value" routine is working. Upto now I have only encountered putting 5v on y+ or x+ and corresponding y- or x- to be 0v. What is 5v @ y+ and 0v and x- doing?
This application note will help you understand better what is going on.
http://www.eetasia.com/ARTICLES/2004...URCES=DOWNLOAD
Before you start reading the touchscreen coordinates, you need to know if the screen has been touched. By having 5V at Y+ and 0V at X- you monitor if the screen was touched. The setup is as follows
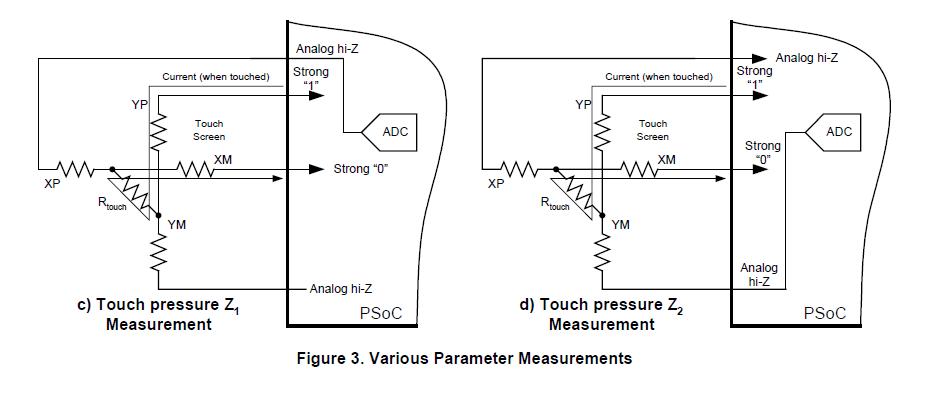
And the code is
Code:
START:
'################ START READING AVERAGE PRESSURE VALUE ################
TRISA = %00001001 ' Set Y+ (portA.2, AN2) and X- (portA.1, AN1) as outputs
' and Y- (portA.3, AN3) and X+ (portA.0, AN0) as inputs
low PORTA.1 ' Set X- = 0V
high PORTA.2 ' Set Y+ = 5V
ADCON0 = %00000001 ' Bits(5-2): 0000=AN0. Start sampling at X+
pauseus 10
ADCON0.1 = 1 ' Stops or continues sampling depending on ADCON2 bits(5-3),
' then begins conversion
GOSUB CONVERSION_ROUTINE ' Obtain value for A/D conversion resolution
PRESSURE_XP = RESOLUTION ' Obtain PRESSURE_XP from X+ sampling, X- = 0V, and Y+ = 5V
ADCON0 = %00001101 ' Bits(5-2): 0011=AN3. Start sampling at Y-
pauseus 10
ADCON0.1 = 1 ' Stops or continues sampling depending on ADCON2 bits(5-3),
' then begins conversion
GOSUB CONVERSION_ROUTINE ' Obtain value for A/D conversion resolution
PRESSURE_YM = RESOLUTION ' Obtain PRESSURE_YM from Y- sampling, X- = 0V, and Y+ = 5V
IF (PRESSURE_XP > 24) AND (PRESSURE_YM < 1000) THEN ' THE TOUCHSCREEN HAVE BEEN TOUCHED
GOSUB READ_COORDINATES
gosub CONVERT_TO_PIXELS
ENDIF
LCDOUT $FE, 1 ' Clear LCD
LCDOUT $FE,2,"X=",DEC5 X_VALUE,", Y=",DEC5 Y_VALUE ' DISPLAYS A/D CONVERSION VALUES
LCDOUT $FE,$C0,"P1=",DEC4 PRESSURE_XP,", P2=",DEC4 PRESSURE_YM
GOTO START
If the screen is not touched then the reading for PRESSURE_XP is going to be very close to zero and the reading for PRESSURE_YM is going to be very close to 1024, remember it is a 10-bit ADC. The screen has been touched when the following condition has been met
Code:
IF (PRESSURE_XP > 24) AND (PRESSURE_YM < 1000) THEN ' THE TOUCHSCREEN HAVE BEEN TOUCHED
To read the X,Y coordinates you need the following setup.
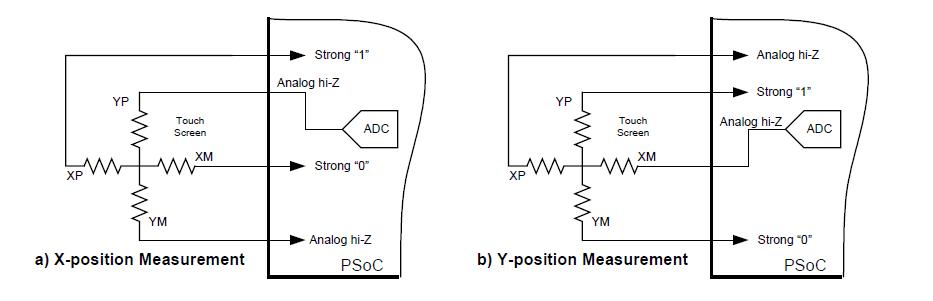
And the code is
Code:
'****************************************************************
'* READ X AND Y VALUES SUBROUTINE *
'****************************************************************
READ_COORDINATES:
' PORTA.0 (AN0) -> X+
' PORTA.1 (AN1) -> X-
' PORTA.2 (AN2) -> Y+
' PORTA.3 (AN3) -> Y-
'################ READ AVERAGE X-VALUE ################
TRISA = %00000011 ' Set X+ (portA.0, AN0) and X- (portA.1, AN1) as inputs
' and Y+ (portA.2, AN2) and Y- (portA.3, AN3) as outputs
low PORTA.3 ' Set Y- = 0V
high PORTA.2 ' Set Y+ = 5V
ADCON0 = %00000001 ' Bits(5-2): 0000=AN0. Start sampling at X+
pauseus 10
ADCON0.1 = 1 ' Stops or continues sampling depending on ADCON2 bits(5-3),
' then begins conversion
GOSUB CONVERSION_ROUTINE ' Obtain value for A/D conversion resolution
X_VALUE = RESOLUTION ' Obtain X_VALUE from X+ sampling, Y- = 0V, and Y+ = 5V
ADCON0 = %00000101 ' Bits(5-2): 0001=AN1. Start sampling at X-
pauseus 10
ADCON0.1 = 1 ' Stops or continues sampling depending on ADCON2 bits(5-3),
' then begins conversion
GOSUB CONVERSION_ROUTINE ' Obtain value for A/D conversion resolution
X_VALUE = (X_VALUE + RESOLUTION) ' Obtain X_VALUE from X- sampling, Y- = 0V, and Y+ = 5V
'################ READ AVERAGE Y-VALUE ################
TRISA = %00001100 ' Set X+ (portA.0, AN0) and X- (portA.1, AN1) as outputs
' and Y+ (portA.2, AN2) and Y- (portA.3, AN3) as inputs
low PORTA.1 ' Set X- = 0V
high PORTA.0 ' Set X+ = 5V
ADCON0 = %00001001 ' Bits(5-2): 0010=AN2. Start sampling at Y+
pauseus 10
ADCON0.1 = 1 ' Stops or continues sampling depending on ADCON2 bits(5-3),
' then begins conversion
GOSUB CONVERSION_ROUTINE ' Obtain value for A/D conversion resolution
Y_VALUE = RESOLUTION ' Obtain Y_VALUE from Y+ sampling, X- = 0V, and X+ = 5V
ADCON0 = %00001101 ' Bits(5-2): 0011=AN3. Start sampling at Y-
pauseus 10
ADCON0.1 = 1 ' Stops or continues sampling depending on ADCON2 bits(5-3),
' then begins conversion
GOSUB CONVERSION_ROUTINE ' Obtain value for A/D conversion resolution
Y_VALUE = (Y_VALUE + RESOLUTION) ' Obtain Y_VALUE from Y- sampling, X- = 0V, and X+ = 5V
RETURN
'****************************************************************
'* CONVERSION SUBROUTINE *
'****************************************************************
CONVERSION_ROUTINE:
REPEAT ' Wait until conversion is finished. When finished ADCON0.1 will be =0
PAUSEUS 10
CONV_STATUS = ADCON0.1
UNTIL CONV_STATUS = 0
RESOLUTION.BYTE0 = ADRESL : RESOLUTION.BYTE1 = ADRESH ' READ A/D CONVERSION VALUE
RETURN
For the final routine "CONVERT_TO_PIXELS" the constants Ax, Bx, Ay, and By should be found experimentally. They will vary depending on the resolution of the LCD screen. One thing to remember is that the relation of the 4-wire touchscreen readings and the corresponding LCD pixels behaves almost linearly.
Code:
'****************************************************************
'* CONVERT_TO_PIXELS ROUTINE *
'****************************************************************
CONVERT_TO_PIXELS:
'Ax, Bx, Ay, and By should be found experimentally
X_VALUE = Ax * X_VALUE + Bx
Y_VALUE = Ay * Y_VALUE + By
RETURN
Bookmarks